In .NET, dynamic filtering can be implemented in various ways depending on the data source and the technology stack you're using (e.g., Entity Framework, LINQ, or a simple in-memory collection).
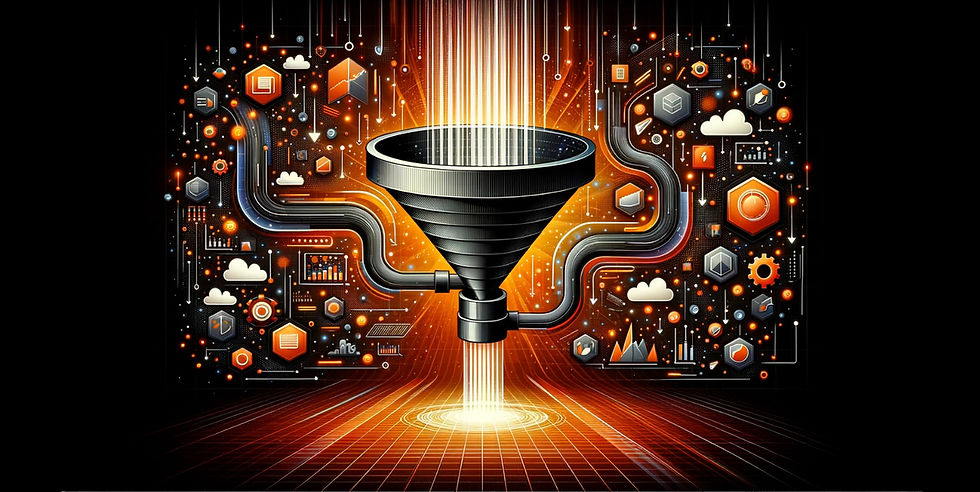
Below are the common steps and approaches to implement dynamic filtering in a typical ASP.NET or .NET Core application, with Entity Framework as an example.
1. Using Dynamic LINQ Queries
You can use LINQ to dynamically build queries that filter data based on user input or form data. This approach is most commonly used when you're dealing with databases through Entity Framework (EF) or in-memory collections.
Example:
Here’s a simple example of dynamic filtering in .NET using LINQ and Entity Framework Core:
In this example:
AsQueryable(): Converts the DbSet to an IQueryable to allow dynamic query building.
Conditional Filters: Each filter is applied only if a corresponding condition is met (e.g., non-null value, non-empty string).
2. Using Dynamic Expressions (Dynamic LINQ)
The Dynamic LINQ library allows you to build LINQ queries dynamically by passing string expressions, which is useful for very flexible, runtime-based filtering.
You can install it via NuGet:
Install-Package System.Linq.Dynamic.Core
Example:
If you pass "Price >= 10 AND Category == 'Electronics'" as the filterExpression, it will dynamically apply this to the query.
3. Using Predicate Builders for Complex Dynamic Filters
The Predicate Builder pattern allows you to dynamically combine filters, especially when building complex queries where multiple filters need to be combined using AND/OR conditions.
First, install the LINQKit library via NuGet:
Install-Package LinqKit
Then, you can use PredicateBuilder to construct dynamic filters:
PredicateBuilder.New<Product>(true): Initializes a predicate that can be dynamically extended.
And/Or: You can chain multiple conditions with And or Or for flexible, complex filtering logic.
4. Dynamic Filtering in ASP.NET MVC or Blazor
When implementing dynamic filtering in ASP.NET MVC or Blazor, user input is typically received through forms, query strings, or model binding. Here’s how you might dynamically filter results in an ASP.NET MVC or Blazor app based on form input.
ASP.NET MVC Example:
In the controller, you accept form data as parameters:
And in your Razor View (or Blazor Component):
The form inputs allow users to dynamically change filters, and the results are updated accordingly after each submission.
5. Dynamic Filtering in Blazor
In a Blazor component, you can achieve dynamic filtering through data binding and event handling:
Conclusion
Dynamic filtering in .NET can be implemented in several ways, depending on the requirements and technology stack. Whether using LINQ, Entity Framework, Dynamic LINQ, or Predicate Builders, the goal is to allow users to dynamically filter large datasets with efficient query handling.
To take your development to the next level, consider using DeliaWeb, a free .net web application database builder. With DeliaWeb, you can easily create and manage dynamic databases without the need for extensive coding. It's designed to streamline your workflow and empower you to build applications faster and more efficiently.
Don’t miss out on this opportunity!
Yorumlar