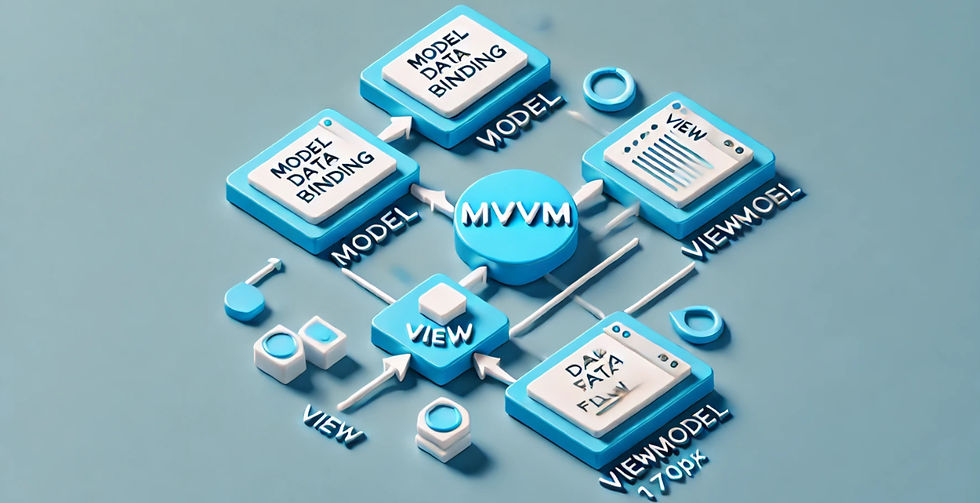
MVVM (Model-View-ViewModel) is a design pattern widely used in UI development to separate concerns. In ASP.NET applications, particularly those that involve rich client-side interactions, it can be implemented using frameworks like Knockout.js or Blazor, which utilize server-side binding. Here's a breakdown of how MVVM works in this context, focusing on server-side binding.
MVVM in ASP.NET: Key Concepts
Model: Represents the data and business logic of the application. In ASP.NET, this could be your data models or domain entities, often tied to database structures or services.
View: This is the UI or the presentation layer, typically built using Razor views, Blazor components, or HTML/JavaScript files in ASP.NET. The view displays the data and interacts with the user.
ViewModel: Acts as an intermediary between the View and Model. It exposes data from the Model to the View in a form that is easily consumable by the UI. The ViewModel also handles input validation, command handling, and other business logic that may be needed by the UI.
Server-Side Binding in ASP.NET
Server-side binding means that data binding occurs between the ViewModel and the View on the server side, unlike client-side frameworks where the ViewModel resides in the browser. In ASP.NET, this can be implemented with technologies like Blazor Server or Razor Pages combined with JavaScript frameworks (e.g., Knockout.js) to handle the binding between the View and ViewModel.
Blazor Server: MVVM with Server-Side Binding
Blazor Server is a powerful way to implement the MVVM pattern with server-side rendering and interaction. In Blazor Server, the ViewModel is a C# class that maintains state and logic on the server, and it communicates with the View through WebSockets. Blazor handles the synchronization of data between the server and the client UI.
Model: The data from the database or API that the application works with.
View: Razor components written in C# and HTML, which are rendered to the user.
ViewModel: The C# classes containing logic that expose properties to the View (via binding) and methods for handling UI events.
How it works:
You define @bind attributes in the Razor components to bind UI elements (inputs, buttons) to the properties of the ViewModel.
The server processes user actions, and the changes are reflected in real-time in the UI through a SignalR connection.
Example:
Here, the button clicks are handled by the server, and the ViewModel (count and IncrementCount) resides in the Razor component.
ASP.NET with Knockout.js: MVVM with Client-Side Binding
In traditional ASP.NET applications (like ASP.NET MVC or Web Forms), Knockout.js can be used to implement MVVM with client-side binding. Knockout provides a way to bind the ViewModel (JavaScript) directly to the View (HTML) and communicate with the server for data operations.
Model: Server-side C# objects (passed to JavaScript through AJAX, JSON, or Razor).
View: HTML view pages or Razor pages.
ViewModel: JavaScript object using Knockout.js observables, computed properties, and bindings.
How it works:
The View is bound to the JavaScript ViewModel using Knockout bindings (data-bind attributes).
The ViewModel is populated from server data using AJAX, and the View reflects changes in real-time as users interact with it.
Example:
Server-side interaction happens through AJAX calls, updating the Model on the server. For example:
Key Differences between Blazor and Knockout.js
Blazor Server relies on server-side processing, and updates are pushed to the UI through a WebSocket connection.
Knockout.js is a client-side MVVM framework, so it runs in the browser and uses AJAX for server communication.
Conclusion
In ASP.NET, the MVVM pattern can be implemented using ViewModel classes on the server side and client-side frameworks like Knockout.js to facilitate two-way data binding and real-time UI updates. This enables a richer, more interactive user experience, similar to what MVVM achieves in WPF, while still adhering to the principles of MVC in ASP.NET.
To take your development to the next level, consider using DeliaWeb, a free .net web application database builder. With DeliaWeb, you can easily create and manage dynamic databases without the need for extensive coding. It's designed to streamline your workflow and empower you to build applications faster and more efficiently.
Don’t miss out on this opportunity!
Comentários